03 Newmeric Type
Here's the translated markdown:
1. Characteristics of Numeric Types
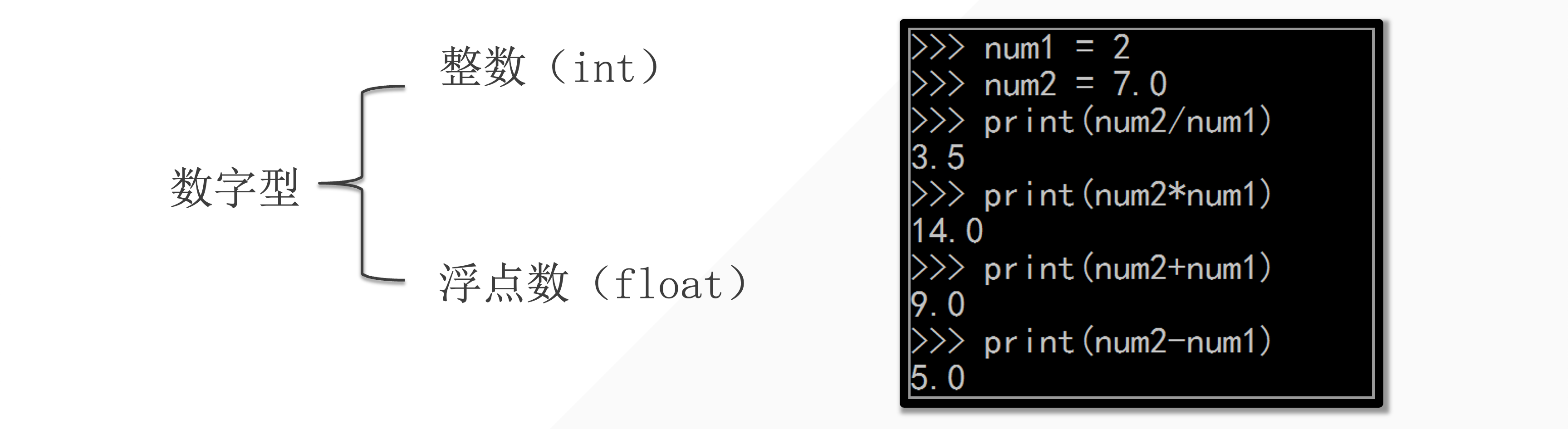
In [3]: 1 + 1
Out[3]: 2
In [4]: 1 + 1.0
Out[4]: 2.0
In [5]: 2 -1
Out[5]: 1
In [6]: 2 - 1.0
Out[6]: 1.0
In [7]: 2 * 2
Out[7]: 4
In [8]: 2* 2.0
Out[8]: 4.0
In [9]: 9/3
Out[9]: 3.0
# The result is a float if one of the elements is a float, with the highest precedence.
# Division involves precision issues, resulting in a float.
2. Arithmetic Operators
Arithmetic Operators: Used for arithmetic calculations.
Operator | Description | Example |
---|---|---|
+ | Addition Operator | 1 + 1 = 2 |
- | Subtraction Operator | 2 - 1 = 1 |
* | Multiplication Operator | 2 * 3 = 6 |
/ | Division Operator | 9 / 3 = 3.0 |
** | Exponentiation Operator | 2 ** 3 = 8 |
% | Modulus Operator, calculates remainder | 9 % 2 = 1 |
// | Floor Division Operator, calculates quotient without remainder | 9 // 2 = 4 |
Tip: 9 / 2 = 4......1
3. Trying It Out: Number Transformation
Imagine you have a two-digit integer, and we need to generate two new numbers based on the following rules:
- The first new number is the sum of the individual digits of the original number.
- The second new number is the reverse of the original number (e.g., for 21, the reversed number is 12).
Write Python code to fulfill these requirements.
Input:
An integer num
(10 ≤ num ≤ 99)
Output:
Two integers or an error message string.
Example:
Suppose the input number num
is 91. Then your code should output two numbers: 10 (the sum of 9 and 1) and 19 (the reversal of 91).
Suppose the input number num
is 26. Then your code should output two numbers: 8 (the sum of 2 and 6) and 62 (the reversal of 26).
Suppose the input number num
is 18. Then your code should output two numbers: 9 (the sum of 1 and 8) and 81 (the reversal of 18).
num = 91
print(num // 10 + num % 10)
print(10 * (num % 10) + num // 10)
#output
10
19
4. Comparison Operators: Comparing Values
Operator | Description | Example |
---|---|---|
> | Checks if the first operand is greater than the second | print(1 > 2) |
< | Checks if the first operand is less than the second | print(1 < 2) |
>= | Checks if the first operand is greater than or equal to the second | print(3 >= 3) |
<= | Checks if the first operand is less than or equal to the second | print(3 <= 4) |
== | Checks if two operands are equal | print(2 == 2) |
!= | Checks if two operands are not equal | print(2 != 1) |
Try the above examples and see the resulting outputs.
print(1 > 2)
print(1 < 2)
print(3 >= 3)
print(3 <= 4)
print(2 == 2)
print(2 != 1)
#output
False
True
True
True
True
True
5. Assignment Operators
Operator | Description | Example |
---|---|---|
= | Assigns the value on the right-hand side to the left-hand side | a = 1 |
+= | a += b is equivalent to a = a + b | a += 10 |
-= | a -= b is equivalent to a = a - b | a -= 10 |
*= | a *= b is equivalent to a = a * b | a *= 10 |
/= | a /= b is equivalent to a = a / b | a /= 10 |
**= | a **= b is equivalent to a = a ** b | a **= 10 |
//= | a //= b is equivalent to a = a // b | a //= 10 |
# Assignment Operators
a = 1
a += 10
print(a)#11
# Regular notation
a = 1
a = a + 10
print(a)#11
7.3.1 Sum and Difference of Numbers:
Write Python code that creates two numbers a
and b
, calculates and prints their sum and the result of a
subtracted from b
.
Code Template
a = 10
b = 12
sum_result = a + b
difference = a - b
print("Sum: ", sum_result)
print("Difference: ", difference)
# Test
assert sum_result == a + b
assert difference == a - b
Output Example:
Sum: 8
Difference: -2
7.3.2 Multiplication and Division of Numbers
Write Python code that creates two numbers x
and y
, calculates and prints their product and the result of x
divided by y
.
Code Template
x = 3
y = 4
print("Product: ", x * y)
print("Division: ", x / y)
# Test
assert product == x * y
assert division == x / y
Output Example:
Product: 12
Division: 0.75
7.3.3 Modulus and Exponentiation
Write Python code that creates two numbers m
and n
, calculates and prints m
modulo n
and m
raised to the power of n
.
Code Template
m = 3
n = 4
print("Modulus: ", m % n)
print("Power: ", m ** n)
# Test
assert remainder == m % n
assert power == m ** n
Output Example:
Modulus: 3
Power: 81
7.3.4 Comparison Operation
Write Python code that takes two numbers p
and q
as input, compares them, and prints the respective comparison result (greater than, less than, equal to).
Code Template
p = int(input("Please enter an integer: "))
q = int(input("Please enter the second integer: "))
if p > q:
print(f"{p} is greater than {q}")
assert p > q
elif p < q:
print(f"{p} is less than {q}")
assert p < q
else:
print(f"{p} is equal to {q}")
assert p == q
Output Example:
- 0
- 0
- 0
- 0
- 0
- 0